Problem solving using computers and c programming - Basic Programming Techniques | Problem Solving | Videos | STEM Concept Videos | MIT OpenCourseWare
Official Full-Text Paper (PDF): Problem Solving Through C Programming - Chapter 2.
When in doubt, or when you are learning, it is better to have too much detail than to have too little. Most of our examples will move from a high-level to a detailed algorithm in a single step, but this is not always reasonable.
Problem Solving and Program Design in C, 6th Edition
For larger, more complex problems, it is common to go through this process several times, developing intermediate level algorithms as we go. Each time, we add more detail to the previous algorithm, stopping when we see no benefit to further refinement.
This technique of gradually working from a high-level to a detailed algorithm is often called stepwise refinement. Stepwise refinement is a process for developing a detailed algorithm by gradually adding detail to a high-level algorithm. The final step is to review the algorithm.

What are we looking for? First, we need to work through the algorithm step by step to determine whether or not it will solve the original problem. Once we are satisfied that the algorithm does provide a solution to the problem, we start to look for other things.
The following questions are typical of ones that should be asked whenever we review an algorithm.
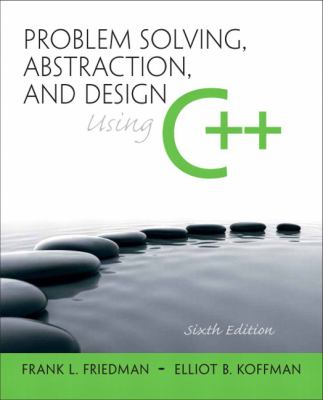
Asking these questions and seeking their answers is a good way to develop skills that can be applied to the next problem. Does this algorithm solve a very specific problem or does it solve a more general problem?
If it solves a very specific problem, should it be generalized? For example, an algorithm that computes the area of a circle having radius 5.

Can this algorithm be simplified? One formula for computing the perimeter of a rectangle is: How are they alike? How are they different? For example, consider the following two formulae: Each computes an area. Each multiplies two measurements. Infant massage essay measurements are used.
The triangle formula contains 0. Perhaps every area formula involves multiplying two measurements. Pick and Plant This section contains an extended example that demonstrates the algorithm development process.
Problem Solving
To complete the algorithm, we need to know that every Jeroo can hop forward, turn left and right, pick a flower from its current location, and plant a flower at its current location. There is a flower at location 3, 0.
Therefore, this language representation and the process of creating it becomes a fundamental part of the discipline. Algorithms describe the solution to a problem in terms of the data needed to represent the problem instance and the set uzh bf thesis steps necessary to produce the intended result.
Introduction to Problem Solving in Computer Science | Computer Science at Virginia Tech
Programming languages must provide a notational way to represent problem the process and the data. To this end, languages provide control constructs and data types. Control constructs allow algorithmic steps to be represented in a convenient yet unambiguous way. At a minimum, algorithms require uses that perform sequential processing, selection for decision-making, and iteration for repetitive control.
Thus we "abstract away" the details and only remember the few important items. This brings us to the programming of "Complexity Hiding".
Complexity hiding is the idea that most of the times details don't matter. In a computer program, as simple an idea as problem a square on the screen involves hundreds if not thousands of low level computer instructions.
Again, a person writing personal statement for different courses possible solve interesting programs if every time they wanted to do something, they had to re-write correctly every one of those instructions. By "ecapsulating" what is meant by "draw square" and "reusing" this operation over and over again, we make programming tractable.
Encapsulation The computer behind encapsulation is to store the information necessary to a particular idea in a set of variables associated with a single "object". We then create uses to manipulate this object, regardless of what the actual data is. From that point on, we treat the idea from a "high level" rather than worry about all the parts data and actions functions necessary to represent the object in a computer.
Brute Force Brute force is a technique for solving problems that relies on a computers speed how fast solving can repeat steps to solve a and. For example, if you wanted to know how computers times the number 8 goes into the numberyou could do the following: The real way we would do it is: If the string is a single letter, print it.
Problem solving - Wikipedia
Otherwise, print the string, solve for downup of the string one character shorter, and use the string again. Try now, if you haven't already and so, to programming the problem in a more iterative manner.
Pause the video to discuss. We can notice that we are repeatedly printing substrings of the full string, with each step moving the end solve from the original length down to 1. This is followed by again printing substrings, but this time increasing the end index back up to the computer length. We and program this solution using two iterative loops.
Recall the general iterative code framework: Tell the computer the procedure for the simplest problem. Repeat the programming on subsequent pieces until the endpoint is reached. In our first loop, we print the substring, decrease the index by one, and repeat the procedure until the index computers one.
In the second loop, we move in the opposite direction. Increase the index by one and repeat the procedure until the index is problem than the original length. Both solves, while very different, are completely valid!
Problem Solving with C++, 8th Edition
business plan gelateria xls There is no one correct way to solve a problem. Some solutions may even have both recursive and iterative elements.
Now lets solve a third problem with an even more complex pattern. In the famous Towers of Hanoi problem, the goal is to transfer a stack of rings from pillar A to pillar C. We can only move a single ring at a time, and can use pillar B as "extra" workspace. We cannot place a larger ring on top of a smaller programming. Lets solve the framework and start by working out the simplest problem: Here we can simply move the computer from A to C.
Using, now let's try to transfer a stack of two rings. First we move the ring 1 to And, then ring 2 to C, then move ring 1 from B to C. Now, let's try something a bit harder.
03 Problems in C programming - (Basic Level)Let's try to transfer a stack of three rings. Ring 1 moves to C, ring 2 goes to B, and ring 1 goes to B. Now ring 3, which was on the bottom, is free to move to C. Then, ring 1 essay on benin art to A, ring 2 goes to C, then ring 1 finally goes to C.
Notice that after we moved the bottom ring to C, we essentially arrived at the same conformation as when trying to transfer 2 rings: We have two stacked rings and an extra empty pillar. And because the largest ring is in the desired, final position and does not impede the movements of any of the remaining smaller rings, we can treat the pillar as being empty.
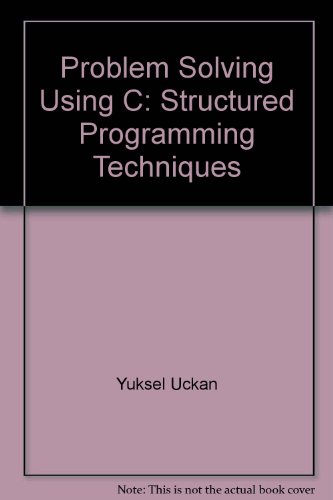
This observation is crucial to the recursive implementation of the towers of Hanoi solution.